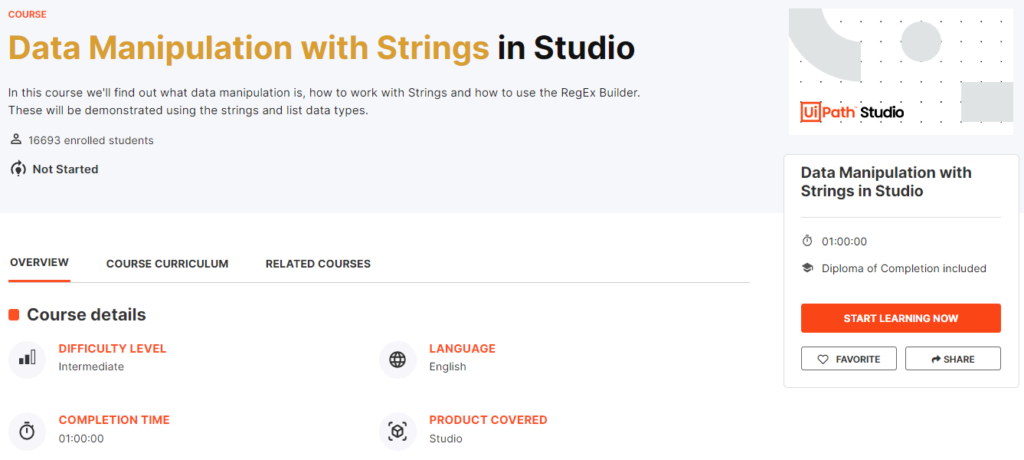
About the course
In this course, you will find out what data manipulation is, how to work with Strings and how to use the RegEx Builder. These will be demonstrated using some of the most common data types that you’ll encounter in your work, namely Strings and Lists.
What you will learn in this course
At the end of this course you will be able to perform the following operations in UiPath Studio:
- Use the most common .NET methods to manipulate values contained in variables of type String.
- Use the RegEx builder in UiPath Studio to perform complex string manipulation.
What is data manipulation?
Data manipulation is the process of modifying, structuring, formatting, or sorting data in order to facilitate its usage and increase its management capabilities.
What are some business scenarios in which I will use data manipulation?
Data manipulation methods are frequently employed by website owners to extract and view specific information from their web server logs. That allows for watching their most popular pages, as well as their traffic sources.
Consider the process of interrogating public financial or legal databases. Data manipulation provides the means for credit analysts to extract only the relevant data and use it in other documents or correlate it with information from other sources.
Objects, Methods and Properties
To understand data manipulation we need to understand what objects, methods and properties are.
Objects are the fundamental building blocks of Visual Basic; almost everything that you do in Visual Basic involves modifying objects. A variable holds an object. For example, if Username = “John Doe”, then the Username variable holds the “John Doe” String object.
Properties are attributes of an object or an aspect of its behaviour. For example, the string class contains two properties: Chars (returns the Char object at a specified position in the current String object) and Length (returns the number of characters in the current String object). For example, based on the previous example, Username.Length returns 8.
Methods are actions that an object can perform. Methods often have parameters that qualify how the action is performed. For example, Username.Replace(“John”,”Jack”) returns “Jack Doe”.
String Methods and Properties
Strings are the data type corresponding to the text. It is difficult to imagine an automation scenario that doesn’t involve the use of strings. Anytime a text needs to be captured, processed, sent between applications, or displayed, strings come in handy (unless the data is structured – like a table).
What are some business scenarios in which you will most likely encounter strings?
- Getting the status of an operation.
- Extracting the relevant piece from a larger text portion.
- Displaying information to the human user.
String.Format
The string Format method is useful to insert the value of a variable or an object or expression into another string. By using the string Format method, we can replace the format items in the specified string with the string representation of specified objects.
The index starts from 0.
Example:
String.Format(“Name:{0} {1}, Location:{2}, Age:{3}”, “Alex”, “Smith”, “Los Angeles”, 32)
Result:
Name: Alex Smith, Location Los Angeles, Age: 32
Working with Date Time Formats
Character | Description | Usage | Example |
---|---|---|---|
d | Short Date | {0:d} | 29-05-2018 |
D | Long Date | {0:D} | 29 May 2018 |
t | Short Time | {0:t} | 05:29:20 |
T | Long Time | {0:T} | 05:29:20 |
f or F | Long Date Time | {0:f} | 29 May 2018 05:30:08 |
g or G | Short Date Time | {0:g} | 29-05-2018 05:31:42 |
M | Short Date | {0:M} | May 29 |
r | RFC1123 Date Time String | {0:r} | Tue, 29 May 2018 05:33:02 GMT |
s | Sortable Date/Time | {0:s} | 2018-05-29T05:34:10 |
u | Universal Sortable Date | {0:u} | 2018-05-29 05:35:47Z |
U | Universal full date | {0:U} | 29 May 2018 00:08:07 |
Y | Year month pattern | {0:Y} | May, 2018 |
Example:
String.Format(“Only Date: {0:D}”, datetime)
Result:
Only Date: 11 October 2022
Working with Number Formats
Character | Description | Usage | Example |
---|---|---|---|
c | Currency | {0:c} | $ 75,674.74 |
e | Scientific | {0:e} | 7.567474e+004 |
f | Fixed Point | {0:f} | 75674.74 |
g | General | {0:g} | 75674.73789621 |
n | Thousand Separator | {0:n} | 75,674.74 |
Example:
String.Format(“Profit: {0:c}”, 14375674.73789621)
Result:
Profit: $ 14,375,674.74 😎
Working with Custom Formats
Character | Description | Usage | Example |
---|---|---|---|
0 | Zero Placeholder | {0:00.00} | 75674.74 |
# | Digit Placeholder | {0:(#).##} | (75674).74 |
. | Decimal Point | {0:0.000} | 75674.738 |
, | Thousand Separator | {0:0,0} | 75,675 |
% | Percent | {0:0%} | 7567474% |
Example:
String.Format(“Profit: {0:0,0}”, 14375674.73789621)
Result:
Profit: 14,375,674
String.Split
The string Split method is useful to split a string into substrings based on the characters in an array. The split method will return a string array that will contain substrings that are delimited by the specified characters in an array.
Example:
Names = “Alex, Mark, John”
Names.Split(“,”c)
Working with Multiple Delimiters
Example:
Names = “Alex, Mark, John, -James%Smith”
Names.Split({“,”c, “-“c, “%”c}, StringSplitOptions.RemoveEmptyEntries)
If you observe the above example, we used a Split() method with multiple delimiters to split the given string into a string array. Here the StringSplitOptions.RemoveEmptyEntries property is useful to remove the empty string elements while returning the result array.
String.Join
The string Join method is useful to concatenate all the elements of a string array using the specified separator between each element.
Example:
Array = {“Welcome”, “to”, “Miami”}
String.Join(” “, Array)
Result:
Welcome to Miami
We used a string Join() method to append or concatenate all the elements of a string array using a specified separator.
String.Trim
The string Trim method is used to remove all leading and trailing whitespace characters from the specified string object in visual basic. Using the Trim() method, we can also remove all occurrences of specified characters from the start and end of the current string.
Example:
Message = ” Hello “
Message.Trim()
String.Contains
The string Contains method is useful to check whether the specified substring exists in the given string or not and it will return a boolean value.
Example:
Message = “Welcome to Miami”
Message.Contains(“Miami”)
String.Concat
The string Concat method is useful to concatenate or append one string to the end of another string and return a new string.
Example:
FirstMessage = “Welcome to Miami”
SecondMessage = “, bienvenido a Miami.”
String.Concat(FirstMessage, SecondMessage)
Result:
Welcome to Miami, bienvenido a Miami.
String.Substring
The Substring method is useful to get a substring from the given string. The substring starts from the specified character position and continues to the end of the string.
The index starts from 0.
Example:
Message = “Welcome to Miami”
Message.Substring(11)
Result:
Miami
*** scroll all the way down to the congrats section. I have a surprise for you😎
String.IndexOf
The string IndexOf method is useful to return the index of the first occurrence of a specified character in the given string.
Example:
Name = “Alex”
Name.IndexOf(“l”)
Result:
1
Constants Class
vbCrLf (similar to pressing Enter)
Represents a carriage-return character combined with a linefeed character for print and display functions.
vbCr (return to the beginning of the line)
Represents a carriage-return character for print and display functions.
vbLf (go to the next line)
Represents a linefeed character for print and display functions.
Other worthy mentions
LastIndexOf, ToList, Copy, ToLower, ToUpper
String Properties
Property | Description |
---|---|
Chars | It will help us to get the characters from the current string object based on the specified position. |
Length | It will return the number of characters in the current String object. |
The RegEx Builder
Regular Expression (REGEX, or regexp) is a specific search pattern that can be used to easily match, locate and manage text. However, creating RegEx expressions may be challenging.
UiPath Studio contains a RegEx builder that simplifies the creation of regular expressions.
Typical uses of RegEx include:
- Input validation.
- String parsing.
- Data scraping.
- String manipulation.
What are some business scenarios in which I will use RegEx?
Retrieving pieces of text that follow a certain pattern, for example:
- extracting phone numbers that start with a certain digit.
- collecting all the street names from a bulk text, even if they don’t follow a specific pattern – some of them contain “Street”, others “Rd.”, and so on.
It would take much longer to build the same expression using the regular String methods – for example, RegEx has a predefined expression to locate all the URLs in a string.
Using the RegEx Builder
The RegEx Builder wizard helps us build and test Regular Expression search criteria.
The Matches activity searches an input string for all occurrences of a regular expression and returns all the successful matches.
The output of the Matches activity is of type IEnumerable of System.Text.RegularExpressions.Match. This is the type we need to set in a For Each activity if we want to iterate through a collection of matches.
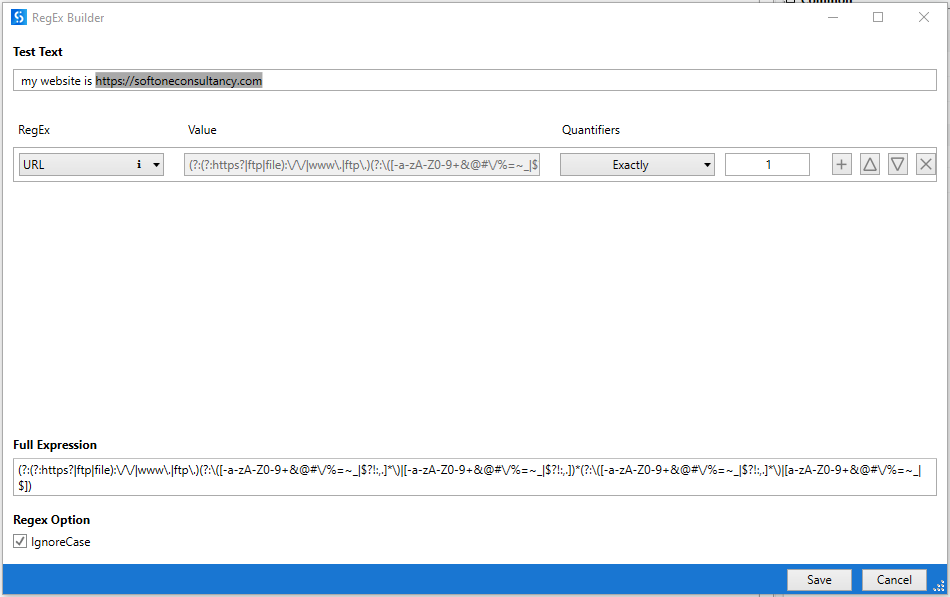
Facts
Four typical uses of RegEx: Input Validation, Data Scraping, String parsing, and String manipulation.
…
Matches: Searches an input string for all occurrences and returns all the successful matches.
IsMatch: Indicates if the specified regular expression finds a match in the specified input string.
Replace: Replaces strings that match a Regex pattern with a specified replacement string.
Congratulation
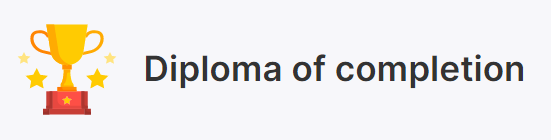
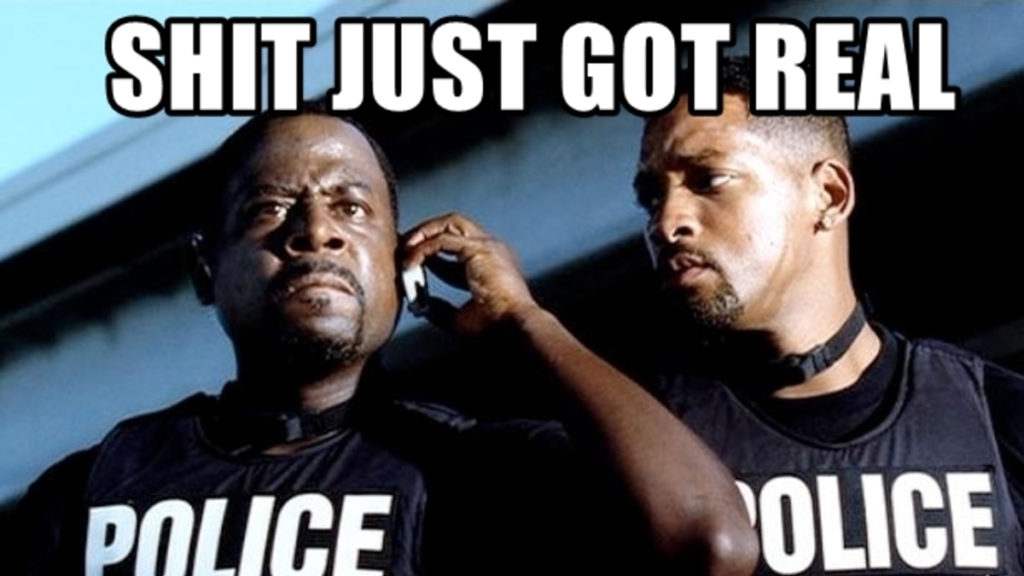