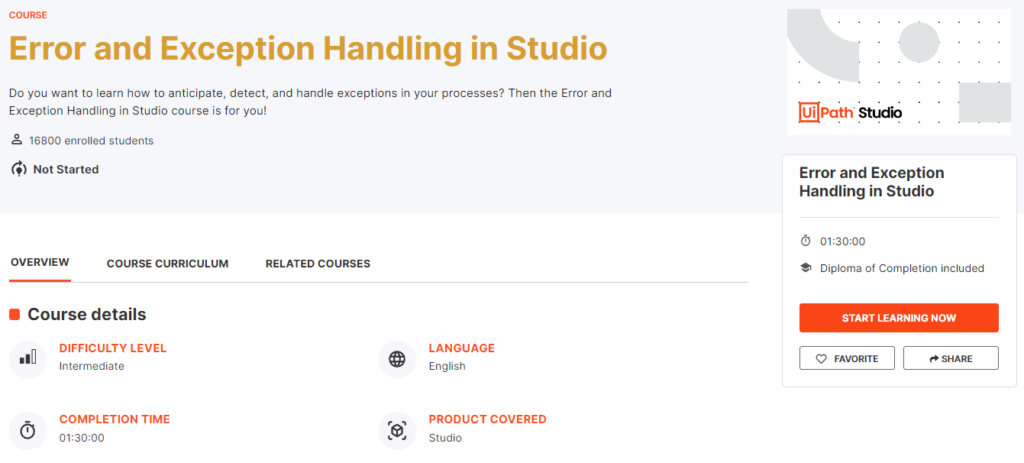
About the course
The Error and Exception Handling in Studio course is an intermediate course that describes exception handling activities like TryCatch, Throw, Rethrow, Retry Scope, and Global Exception Handler. You’ll also learn about a property that UI automation activities share and that is very valuable – ContinueOnError.
What you will learn in this course
At the end of this course you should be able to:
- Identify and differentiate between the different types of exceptions.
- Describe the common exception-handling techniques and explain when they should be used.
- Use the TryCatch, Throw, and Rethrow activities in your automation projects.
- Use the Retry Scope activities in your automation projects.
- Describe the ContinueOnError Property.
- Use the Global Exception Handler in both attended and unattended scenarios.
System and Business Exceptions
Errors are events that a particular program can’t normally deal with. There are different types of errors, based on what’s causing them – for example:
- Syntax errors, where the compiler/interpreter cannot parse the written code into meaningful computer instructions.
- User errors, where the software determines that the user’s input is not acceptable for some reason.
- Programming errors, where the program contains no syntax errors but does not produce the expected results. These types of errors are often called bugs.
Exceptions are events that are recognized (caught) by the program, categorized, and handled. More specifically, there is a routine configured by the developer that is activated when an exception is caught. Sometimes, the handling mechanism can simply stop the execution. Some of the exceptions are linked to the systems used, while others are linked to the logic of the business process.
System exceptions
As a general note, all of these exception types mentioned below are derived from System.Exception, so using this generic type in a TryCatch, for example, will catch all types of errors.
- NullReferenceException – Occurs when using a variable with no set value (not initialized).
- IndexOutOfRangeException – This occurs when the index of an object is out of the limits of the collection.
- ArgumentException – Is thrown when a method is invoked and at least one of the passed arguments does not meet the parameter specification of the called method.
- SelectorNotFoundException – Is thrown when the robot is unable to find the designated selector for an activity in the target app within the TimeOut period.
- ImageOperationException – Occurs when an image is not found within the TimeOut period.
- TextNotFoundException – Occurs when the indicated text is not found within the TimeOut period.
- ApplicationException – Describes an error rooted in a technical issue, such as an application that is not responding.
Business exceptions
A business exception mainly refers to information used in an automated process. Either it may be incomplete or incorrect from a business perspective. When business exceptions occur, robots stop the process, and it requires human intervention to address a thrown exception.
Some of the examples of business exceptions are: certain pieces of data that the automation project depends on are incomplete, missing, outside set boundaries (like trying to extract more from the ATM than its daily limit), or don’t pass other data validation criteria.
Business rule exceptions aren’t automatically generated as system exceptions. They must be defined by a developer by using the Throw Activity and handled inside a TryCatch.
TryCatch, Throw and Rethrow
TryCatch activity catches a specified exception type in a sequence or activity and either display an error notification or dismisses it and continues the execution.
As a mechanism, TryCatch runs the activities in the Try block and, if an error takes place, executes the activities in the Catches block. You can add multiple exceptions and corresponding activities to this block.
The Finally block is only executed when no exceptions are thrown or when an exception is caught and handled in the Catches block (without being re-thrown).
Retry Scope
The Retry Scope activity retries the contained activities as long as the condition is not met or an error is thrown. This activity is used for catching and handling an error, which is why it’s similar to TryCatch. The difference is that this activity simply retries the execution instead of providing a more complex handling mechanism.
The activity has two main sections Actions and Conditions. It can be used without a termination condition, in which case it’ll retry the activities until no exception occurs or the provided number of attempts is exceeded. Retry scope has two additional properties NumberOfRetries (by default is 3) and RetryInterval (by default is 5 seconds).
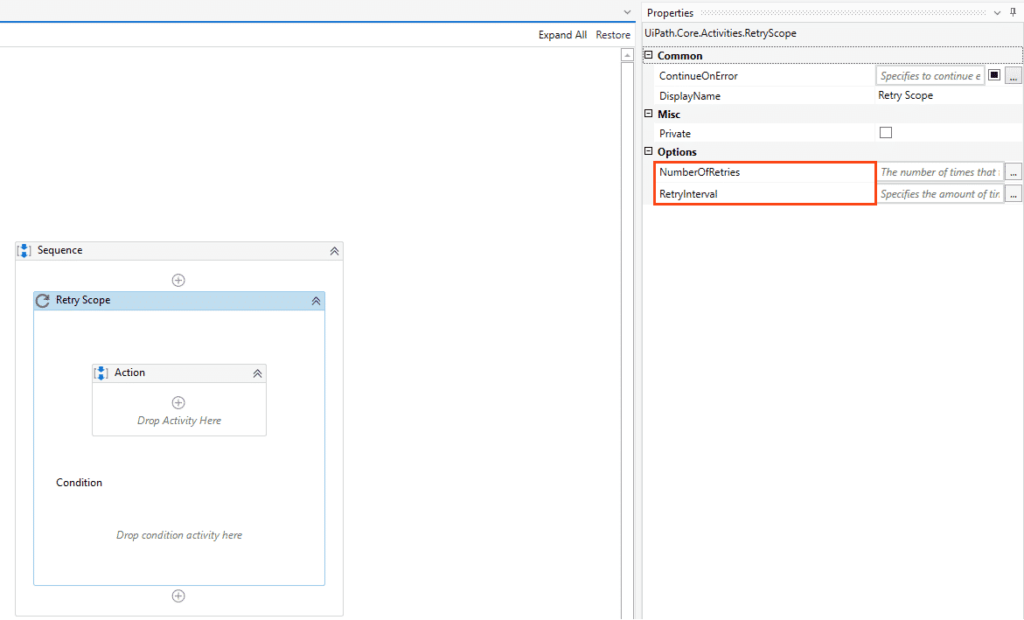
The retry scope performs error handling within the action section. If there’s an error, it’ll just stop wherever the error comes. It’ll wait for the duration that’s set. Otherwise, it’ll wait five seconds and then retry and consume that reading.
NumberOfRetries is just the maximum number of an action sequence that can run. If the NumberOfRetries is set to two, it’ll only retry once, so that the action executes a total of two times.
RetryInterval specifies the amount of time between each retry.
The condition can have zero or one activity, if the condition checked is False, there’ll be another Retry. If the condition checked is True, there won’t be another retry.
The ContinueOnError Property
The ContinueOnError is a property that specifies if the execution of the activity should continue even when the activity throws an error.
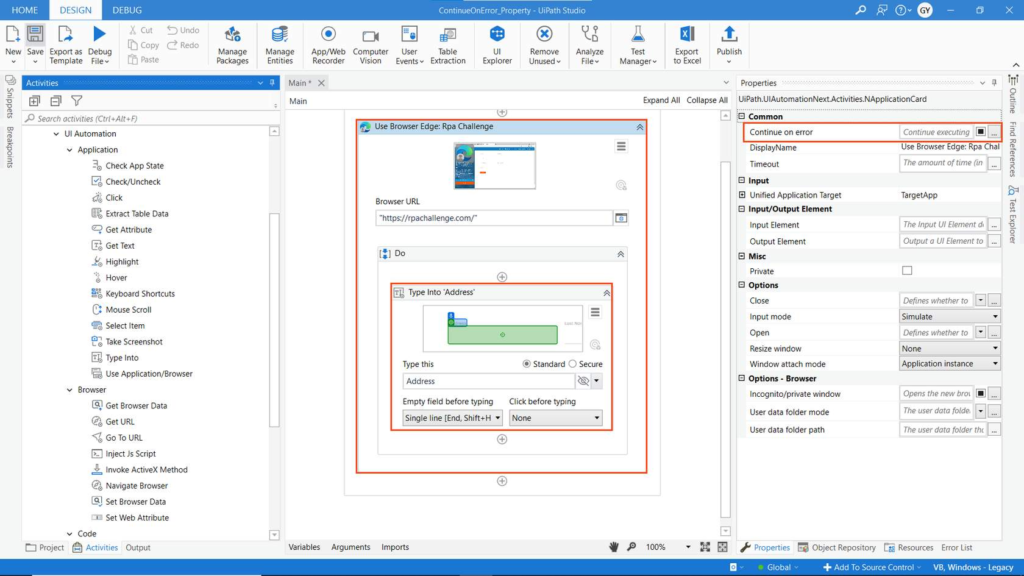
This field only supports Boolean values (True, False). The default value is False, thus, if the field is blank and an error is thrown, the execution of the project stops. If the value is set to True, the execution of the project continues regardless of any error.
Keep in mind that, if the ContinueOnError is set to True on an Activity that has a scope such as (Attach Window or Attach Browser, in the classic, and Use Application Browser in the modern), then any error thrown by any of the activities inside the DO container of respective scope Activity will also be ignored.
Setting this property to True is not recommended in all situations. But there are some in which it makes sense, such as:
- While using data scraping – So that the activity doesn’t throw an error on the last page when the selector of the ‘Next’ button is no longer found.
- When we are not interested in capturing the error but simply in the execution of the activity.
If an activity is included in Try Catch, in the Try section, and the value of the ContinueOnError property is True, no error is caught when the project is executed.
The Global Exception Handler
The Global Exception Handler is a type of workflow designed to determine the process’ behaviour when encountering an unexpected exception. This is why only one Global Exception Handler can be set per automation project.
In its default configuration, the Global Handler catches the exceptions thrown by any activity in the process at runtime and executes a standard response – ignore, retry, abort or continue, as predefined at design time. For attended scenarios, it can be configured to let the user select the action.
A Global Exception Handler can be created by adding a new workflow file with this type. It can also be created by setting an existing workflow as Global Exception Handler from the Project panel.
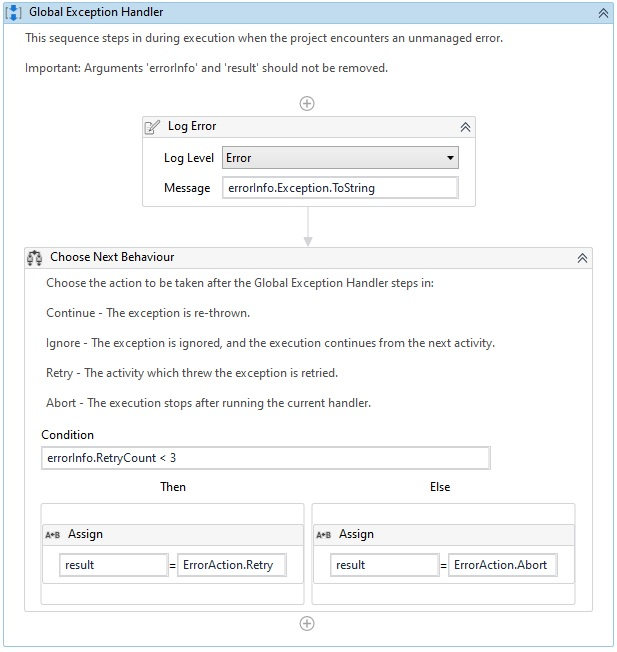
How does it work?
The Global Exception Handler has 2 predefined arguments, that shouldn’t be removed:
- errorInfo with the In direction – contains the information about the error that was thrown and the workflow that failed.
- result with the Out direction – used for determining the next behaviour of the process when it encounters the error.
The Global Exception Handler contains the predefined activities below. If required, we can also add other activities in our Handler.
Log Error: This part simply logs the error. The developer gets to choose the logging level – Fatal, Error, Warn, Info, Trace, and so on.
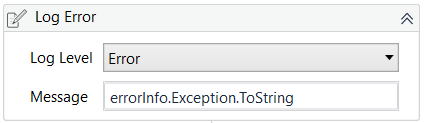
Choose Next Behaviour: Here the developer can choose the action to be taken when an error is encountered during execution:
- Continue – The exception is re-thrown.
- Ignore – The exception is ignored, and the execution continues from the next activity.
- Retry – The activity which threw the exception is retried.
- Abort – The execution stops after running the current handler.
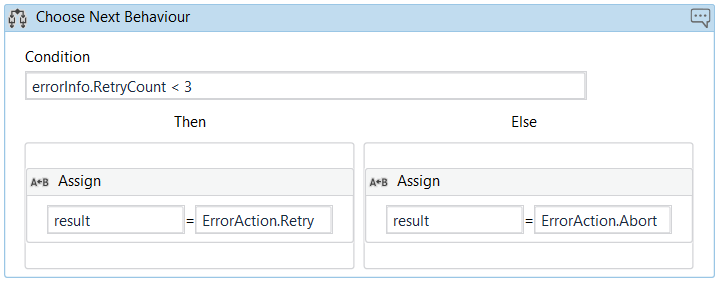
The Global Exception Handler is not available for library projects, only processes.
Only uncaught exceptions will reach the exception handler. If an exception occurs inside a TryCatch activity and is successfully caught and handled inside the Catches block (and not re-thrown), it will not reach the Global Exception Handler.
Facts
Use Ctrl+T to surround an activity with the TryCatch block.
…
What is the limit as to how many Catches you can use in a Try Catch activity?
Answer: No limit
…
In which of the following two cases does the Retry Scope retry the contained activities?
Answer: As long as an error is thrown and As long as the condition is not met.
…
What happens if the ContinueOnError Property is set to True?
Answer: The execution of the project continues regardless of any error.
…
The Global Handler can be set from the Project panel by selecting the XAML file and right-clicking > Set as Global Handler.
Or from the top menu New > Global Handler.
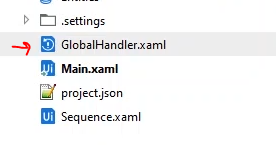
…
To disable the Global Handler select the XAML file and right-click > Remove Handler.
…
The Global Exception Handler has two arguments, errorInfo and result. What are their directions?
Answer: errorInfo has IN direction and the result has an OUT direction.
…
What can you use to make sure that execution continues even if an Activity fails?
Answer: Surround the activity with Try/Catch Activity
…
What is the most effective way to handle the click on a UI Element that is not always available?
Answer: Place the Click activity inside a Try/Catch block.
…
You have more than one exception type defined in the Catch block and an exception occurs that fits two types. Which one of the following blocks is executed?
Answer: The block with most specific match.
…
The Global Exception Handler is designed to determine the project’s behaviour when encountering an execution error. What is a Global Exception Handler?
Answer: a type of workflow.
…
To use a selector in the selector property of an activity, you can store it in a variable. What’s the variable type?
Answer: String
…
Which of the following is recommended to have in a Catch block?
Answer:
- An alternative to the approach that fails
- A LogMessage activity
…
How many Global Exception Handlers can be set per automation project?
Answer: ONE
…
The Retry Scope activity can be used without a termination condition. What does this infer?
Answer: The Retry Scope Activity retries the activities until no exception occurs (or the provided number of attempts is exceeded).
Congratulation
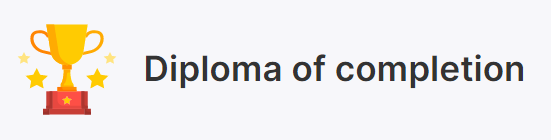
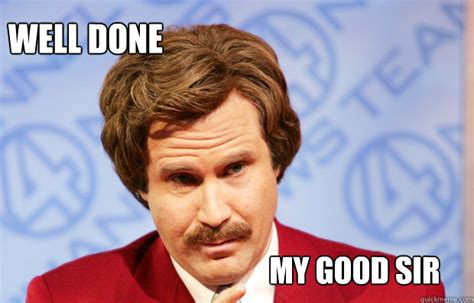